Internet Connection Monitoring
Ensuring a stable and reliable internet connection is essential for any server or system. Network downtime or interruptions can lead to loss of productivity and affect system operations. In this post, we will present a solution for monitoring your internet connection on Ubuntu.
Purpose of the Script
The Ubuntu Internet Connection Monitoring Script monitors the reliability of my internet connection and logs any issues with the connection (it is possible to create a log file and dend it per Email). It tracks lost pings and records when three consecutive pings fail. At the start, you can modify variables to customize the script for your needs. You can also set the log file location.
The MAX_LOST_PINGS
variable defines the threshold for lost pings. Once reached, the log will display: “We reached the threshold of losing $MAX_LOST_PINGS
pings in a row.”
When the internet restores, the script logs: “Ping to 8.8.8.8 is OK” with the exact time.
This should improve the SEO score by making the content clearer and more direct!
Example of the Output of Internet Monitoring Script
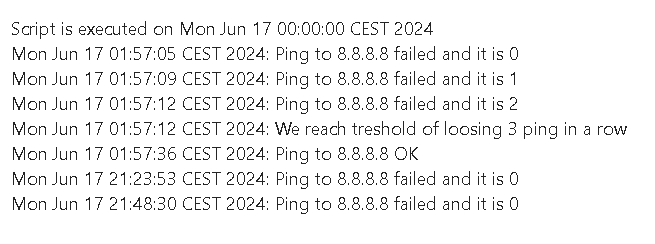
Bash Script Implementation
Create a script with the following command:
nano /opt/scripts/check_internet.sh
Make it executable:
chmod +x /opt/scripts/check_internet.sh
Content of the file:
#!/bin/bash
PING_IP="8.8.8.8" # IP to ping
MAX_LOST_PINGS=3 # Number of lost pings before restarting service
LOG_FILE="/opt/scripts/log/check_internet_$(date +%Y-%m-%d).log" # Location for log file
echo "Check connectivity script is executed on $(date)" >> $LOG_FILE
lost_pings=0 # Define variable to enable tracking lost pings
# Loop indefinitely
while :
do
# Ping the IP once, wait for up to 1 second
if ping -c 1 -W 1 $PING_IP > /dev/null; then
echo "Ping to $PING_IP OK"
if [ $lost_pings -ge $MAX_LOST_PINGS ]; then
echo "$(date): Ping to $PING_IP OK" >> $LOG_FILE
fi
lost_pings=0
else
# Ping failed, log the date, time, and IP
if [ $lost_pings -lt $MAX_LOST_PINGS ]; then
echo "$(date): Ping to $PING_IP failed and it is $lost_pings" >> $LOG_FILE
fi
# Count the number of consecutive lost pings
lost_pings=$((lost_pings + 1))
# If the number of lost pings has reached the limit, restart the service
if [ $lost_pings -eq $MAX_LOST_PINGS ]; then
echo "$(date): We reach treshold of loosing $MAX_LOST_PINGS ping in a row" >> $LOG_FILE
fi
fi
# Wait for 3 seconds before pinging again
sleep 3
# Check if the date has changed
if [ "$(date +%Y-%m-%d)" != "$(date -r "$LOG_FILE" +%Y-%m-%d)" ]; then
# Create a new log file for the new day
LOG_FILE="/opt/scripts/log/check_internet_$(date +%Y-%m-%d).log"
echo "Script is executed on $(date)" >> "$LOG_FILE"
fi
done
Variables
- PING_IP: This is the IP address (8.8.8.8) to ping, which can be modified if needed.
- MAX_LOST_PINGS: This defines how many consecutive pings need to fail before taking action (in this case, the script logs that the threshold is reached).
- LOG_FILE: The location for the log file. It uses the current date to create a unique log file for each day.
Script Breakdown
- Initial Log Entry:
- The script logs the date and time when it’s executed initially (
Check connectivity script is executed on $(date)
), appending it to the log file.
- The script logs the date and time when it’s executed initially (
- Infinite Loop (
while :
):- The script will run forever, constantly pinging the specified IP every 3 seconds.
- Ping Check:
- Inside the loop, it tries to ping the IP address (
$PING_IP
) once with a timeout of 1 second.- If the ping is successful (
ping -c 1 -W 1
), it checks if the number of lost pings has reached the threshold ($MAX_LOST_PINGS
), and if so, logs a success message. - If the ping fails, it logs the failure along with how many consecutive pings have failed.
- If the ping is successful (
- Inside the loop, it tries to ping the IP address (
- Counting Lost Pings:
- Each time a ping fails, it increments a counter (
lost_pings
). Once the count reaches the threshold ($MAX_LOST_PINGS
), the script logs the threshold reach but doesn’t yet act.
- Each time a ping fails, it increments a counter (
- Log Rotation:
- Every time the script checks the internet, it checks if the date has changed since the last log entry. If the date has changed, it creates a new log file for that day to organize logs by date. The old log file continues to log until midnight, then a fresh file starts.
- Sleep:
- After each ping, the script pauses for 3 seconds (
sleep 3
) before the next attempt.
- After each ping, the script pauses for 3 seconds (
Key Actions
- Logging: The script continuously logs whether the ping succeeded or failed.
- Log File Management: It rotates the log file every day.
- Threshold Check: When the number of failed pings reaches the defined threshold, the script logs that the threshold is met.
Install script in the Crontab
If you like to run this script every time after reboot the server, add the following lines in the crontab:
# Check if internet is working
@reboot sleep 180 && sudo bash /opt/scripts/check_internet.sh